Overview
MidiExternalPlay is a prefab included in the Pro version that can play MIDI music from a MIDI file, which is not part of the MPTK-defined MIDI database which is a read-only resource created during the build process.
You app will be able to play a MIDI:
- From a file in a folder anywhere on the device.
- From a web site with a URL.
- From a byte array (by script).
Some points:
- This prefab is available with MPTK Pro.
- Using this prefab does not require any scripting. All tasks can be accomplished through the inspector.
API
For more advanced requirements in your application, an API is usually available. A full example with source code can be found at the bottom of this page.
Important note: The MidiExternalPlay class inherits from both the MidiFilePlayer and MidiSynth classes. Consequently, all attributes and methods from these classes are accessible through MidiExternalPlay.
Below are some quick examples:
Play from a MIDI on a web site:
midiExternalPlayer.MPTK_MidiName = "http://www.midiworld.com/midis/other/c2/bolero.mid";
midiExternalPlayer.MPTK_Play();
Play from a byte array:
using (Stream fsMidi = new FileStream("C:\xxx\Midi\DreamOn.mid", FileMode.Open, FileAccess.Read))
{
byte[] data = new byte[fsMidi.Length];
fsMidi.Read(data, 0, (int)fsMidi.Length);
midiExternalPlayer.MPTK_Play(data);
}
Play Loop inside an external MIDI
When calling MPTK_Play, the MIDI player resets some settings, it’s mandatory to change these settings according to your needs when the MIDI is ready to play. Use the OnEventStartPlayMidi to do this.
MidiExternalPlayer midiExternalPlayer;
private void Start()
{
midiExternalPlayer = FindObjectOfType<MidiExternalPlayer>();
if (midiExternalPlayer != null)
{
// Event trigger when midi file start playing
midiExternalPlayer.OnEventStartPlayMidi.AddListener(StartPlay);
midiExternalPlayer.MPTK_MidiName = yourUri;
midiExternalPlayer.MPTK_Play();
}
}
public void StartPlay(string midiname)
{
Debug.Log("Start Midi " + midiname);
Debug.Log("Duration: " + midiExternalPlayer.MPTK_Duration.TotalSeconds);
midiExternalPlayer.MPTK_InnerLoop.Enabled = true;
midiExternalPlayer.MPTK_InnerLoop.Start = 10000;
midiExternalPlayer.MPTK_InnerLoop.Resume = 10000;
midiExternalPlayer.MPTK_InnerLoop.End = 10200;
midiExternalPlayer.MPTK_InnerLoop.Log = true;
}
Add the prefab in your scene
Before scripting with MidiExternalPlay, it is necessary to add a MidiExternalPlay prefab to your scene.
Inspector parameters
When running:
- Midi URL or file path Example of path regarding the OS:
- Windows
file://C:/Users/Thierry/Desktop/Midi/WishYouWereHere.mid
- Mac
file:///Users/thierry/Desktop/Nirvana.mid
- Web:
http://www.midiworld.com/midis/other/bach/bwv1060b.mid
- Or use the browser icon (on left) to select a file from your desktop.
- Windows
Others Foldout
The inspector inherits of all properties of the MidiFilePlayer inspector, see below for a detailed explanation of others properties .
- Foldout Midi Parameters See here
- Foldout Events See here
- Foldout Midi Info See here
- Synth Parameters See here
- Default Editor See here
Available Demo
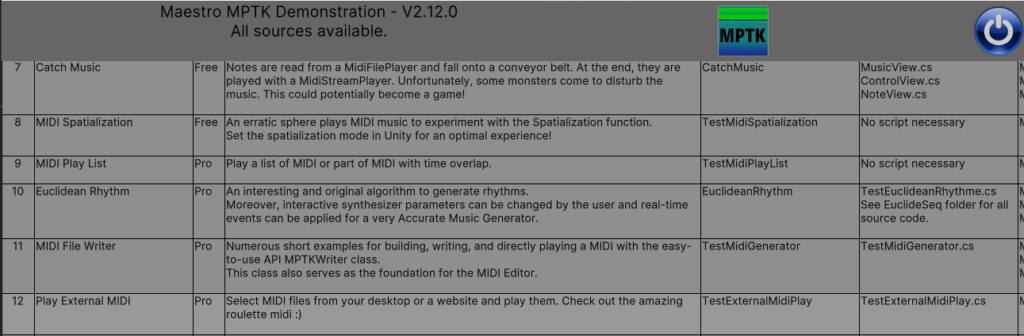
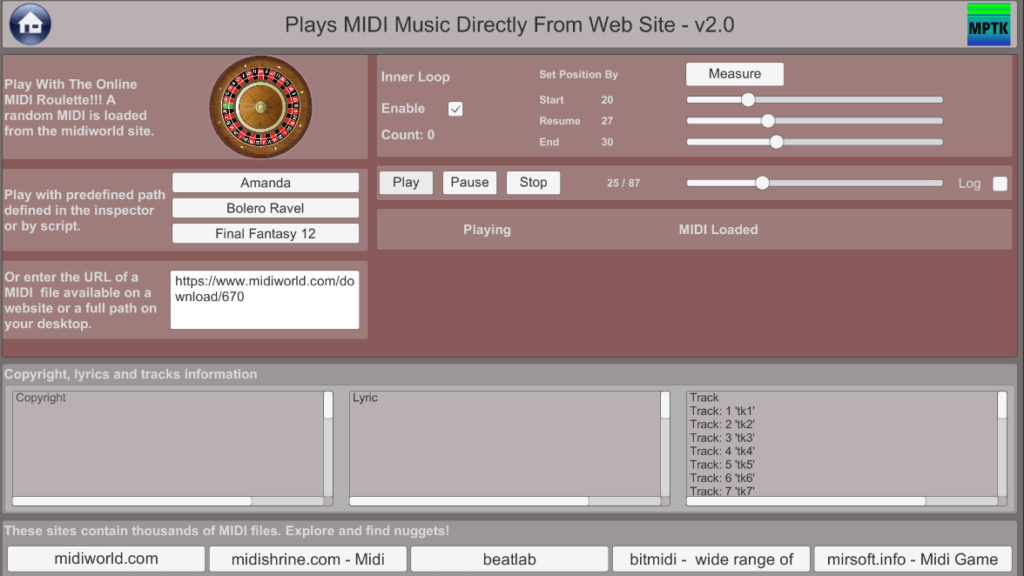
Integration of MidiExternalPlayer in your script
See the TestMidiExternalPlayer.cs source code and the associated events in the TestExternalMidiPlay scene’s GameObjects canvas for the full example.