Please, see our overview page for general information about Maestro Midi Player Tool Kit.
MidiStreamPlayer is a prefab that is perfect for creating MIDI music without a MIDI file: you generate MIDI events from your code and send them to MidiStreamPlayer.
With MidiStreamPlayer you don’t have to add Midi to MPTK but a SoudFont is always needed … to play sound!
You will need at least beginner-level skills to:
- Understand basic MIDI concept.
- The MPTK class MPTKEvent is central to creating MIDI music.
- Writing C# script.
See below how to use this prefab in a few steps:
First, add the prefab to your scene:
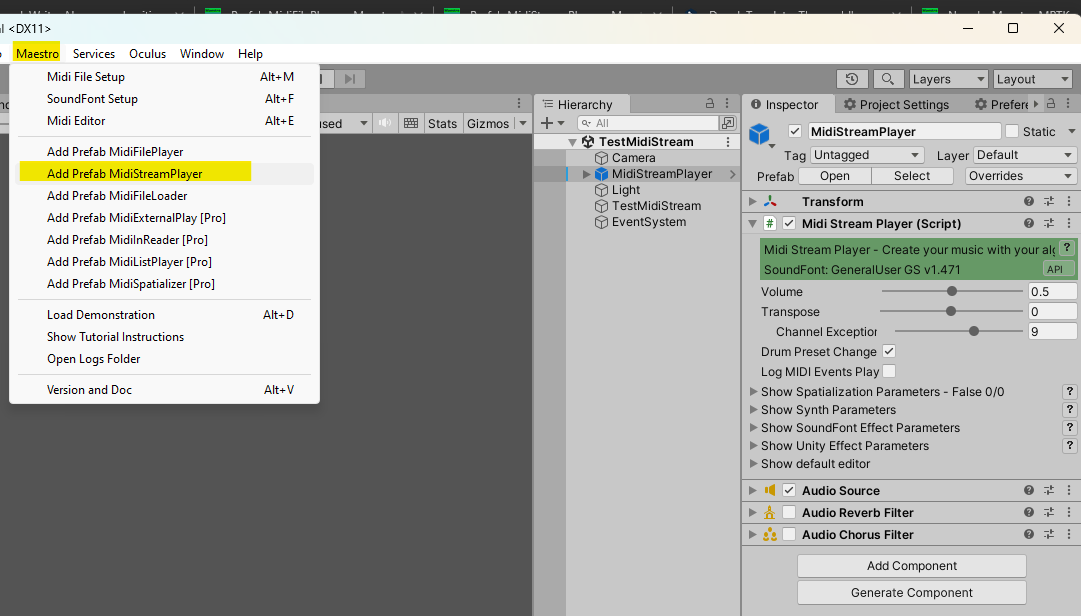
See an example with source code at the bottom of this page.
Secondly, create a midiStreamPlayer variable in your script:
using MidiPlayerTK;
...
// MPTK component able to play a stream of midi events
// Need to be public to be visible in the inspector
public MidiStreamPlayer midiStreamPlayer;
Code language: PHP (php)
Thirdly, use the Unity Inspector to set the reference to the midiStreamPlayer variable created in your script. In this demo, we drag the MidiStreamPlayer game component to the inspector “Midi Stream Player” variable.
That can also be done in your script with FindObjectOfType<MidiStreamPlayer>(). Look at the source code at the bottom.
Now you can call a method in your script to play notes!
The main methods are MPTK_PlayEvent(MPTKEvent evnt)
and MPTK_StopEvent(MPTKEvent evnt)
.
Example:
// Play a C4 for 1 seconds
NotePlaying = new MPTKEvent()
{
Command = MPTKCommand.NoteOn, // midi command
Value = 48, // from 0 to 127, 48 for C4, 60 for C5, ...
Channel = 0, // from 0 to 15, 9 reserved for drum
Duration = 1000, // note duration in millisecond, -1 to play undefinitely, MPTK_StopChord to stop
Velocity = 100, // from 0 to 127, sound can vary depending on the velocity
Delay = 0, // delay in millisecond before playing the note
};
midiStreamPlayer.MPTK_PlayEvent(NotePlaying);
Code language: JavaScript (javascript)
Don’t miss the full description of the MPTKEvent class.
Inspector parameters
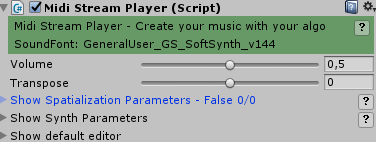
- Volume: set the default volume of the playing.
- Transpose: transpose the music by half tone.
- Show Spatialization Parameters: See here
- Show Synth Parameters: See here
- Show Effect Parameters: See here (available with Pro version, disabled by default)
- Default Editor: See here
As usual, you can modify these parameters by script with the MPTK API.
Example of script with MidiStreamPlayer
See TestMidiStream.cs from MPTK asset (available also in free version) for the full working source code.
See also this guide how to write script with MPTK.
using MidiPlayerTK;<br>...<br>// MPTK component able to play a stream of midi events<br>// Need to be public to be visible in the inspector<br>public MidiStreamPlayer midiStreamPlayer;<br>...<br>// The reference can be set with the inspector or in your script<br>// Search a MidiStreamPlayer in the scene and initialize your variable:<br>midiStreamPlayer = FindObjectOfType<MidiStreamPlayer>();<br>if (midiStreamPlayer == null)<br> Debug.LogWarning("Can't find a MidiStreamPlayer Prefab in the current Scene Hierarchy. Add it with the MPTK menu.");<br><br>if (midiStreamPlayer != null)<br>{<br> if (!midiStreamPlayer.OnEventSynthStarted.HasEvent())<br> // The method EndLoadingSynth will be called when the synth is ready<br> midiStreamPlayer.OnEventSynthStarted.AddListener(EndLoadingSynth);<br>}<br>else<br> Debug.LogWarning("No Stream Midi Player associed to this game object");<br><br>public void EndLoadingSynth(string name)<br>{<br> // Change the default instrument when synth is started.<br> // It's done also with a MIDI event and the command = MPTKCommand.PatchChange<br> midiStreamPlayer.MPTK_PlayEvent(<br> new MPTKEvent() <br> { <br> Command = MPTKCommand.PatchChange, <br> Value = CurrentPatchInstrument, <br> Channel = StreamChannel, <br> });<br>}<br><br>private void BankPatchChanged(object tag, int index)<br>{<br> switch ((string)tag)<br> {<br> case "BANK_INST":<br> midiStreamPlayer.MPTK_PlayEvent(new MPTKEvent()<br> { <br> Command = MPTKCommand.ControlChange, <br> Controller = MPTKController.BankSelect, <br> Value = index, <br> Channel = StreamChannel,<br> });<br> break;<br><br> case "PATCH_INST":<br> midiStreamPlayer.MPTK_PlayEvent(new MPTKEvent()<br> { <br> Command = MPTKCommand.PatchChange, <br> Value = index, <br> Channel = StreamChannel, <br> });<br> break;<br>}<br><br>void Update()<br>{<br> ... <br> // Start playing a new note<br> NotePlaying = new MPTKEvent()<br> {<br> Command = MPTKCommand.NoteOn,<br> Value = CurrentNote,<br> Channel = StreamChannel,<br> Duration = 9999999, // 9999 seconds but stop by the new note. See before.<br> Velocity = Velocity // Sound can vary depending on the velocity<br> };<br> midiStreamPlayer.MPTK_PlayEvent(NotePlaying);<br><br> ... <br><br> if (NotePlaying != null)<br> {<br> // Stop the note (method to simulate a real human on a keyboard : duration is not known when note is triggered)<br> midiStreamPlayer.MPTK_StopEvent(NotePlaying);<br> NotePlaying = null;<br> }<br>}
Code language: HTML, XML (xml)
Have Fun!